ESP32 HTE501 Temperature and Humidity Sensor
The E+E HTE501 is a digital humidity and temperature sensor designed for high accuracy in demanding environments. Compactly housed in a DFN package (2.5x2.5 mm), it offers ±1.8% RH and ±0.2 °C accuracy, and features a constant current heater and proprietary coating for stability.
Jump to Code Examples
Quick Links
HTE501 Sensor Technical Specifications
Below you can see the HTE501 Temperature and Humidity Sensor Technical Specifications. The sensor is compatible with the ESP32, operating within a voltage range suitable for microcontrollers. For precise details about its features, specifications, and usage, refer to the sensor’s datasheet.
- Protocol: I2C
- Interface: I2C, 8-pin DFN
- Accuracy: ±1.8% RH, ±0.2 °C
- Operating Range: -40 to +135 °C, 0–100% RH
- Voltage: 2.35 – 3.60V
HTE501 Sensor Pinout
Below you can see the pinout for the HTE501 Temperature and Humidity Sensor. The VCC
pin is used to supply power to the sensor, and it typically requires 3.3V or 5V (refer to the datasheet for specific voltage requirements). The GND
pin is the ground connection and must be connected to the ground of your ESP32!
HTE501 Wiring with ESP32
Below you can see the wiring for the HTE501 Temperature and Humidity Sensor with the ESP32. Connect the VCC pin of the sensor to the 3.3V pin on the ESP32 or external power supply for power and the GND pin of the sensor to the GND pin of the ESP32. Depending on the communication protocol of the sensor (e.g., I2C, SPI, UART, or analog), connect the appropriate data and clock or signal pins to compatible GPIO pins on the ESP32, as shown below in the wiring diagram.
Code Examples
Below you can find code examples of HTE501 Temperature and Humidity Sensor with ESP32 in several frameworks:
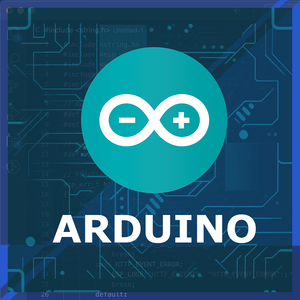
ESP32 HTE501 Arduino IDE Code Example
Fill in your main
Arduino IDE sketch file with the following code to use the HTE501 Temperature and Humidity Sensor:
#include <Wire.h>
#include <Adafruit_SHT31.h> // Adafruit library compatible with I2C humidity/temp sensors
Adafruit_SHT31 sht31 = Adafruit_SHT31();
void setup() {
Serial.begin(115200); // Start the serial communication
Wire.begin(); // Initialize I2C
// Initialize the sensor
if (!sht31.begin(0x44)) { // The default I2C address for many SHT3x sensors is 0x44
Serial.println("Couldn't find HTE501 sensor!");
while (1) delay(1); // Stop if the sensor is not found
}
Serial.println("HTE501 Sensor initialized.");
}
void loop() {
float temperature = sht31.readTemperature(); // Read temperature in Celsius
float humidity = sht31.readHumidity(); // Read relative humidity in %
if (!isnan(temperature)) { // Check if reading is valid
Serial.print("Temperature: ");
Serial.print(temperature);
Serial.println(" °C");
} else {
Serial.println("Error reading temperature.");
}
if (!isnan(humidity)) { // Check if reading is valid
Serial.print("Humidity: ");
Serial.print(humidity);
Serial.println(" %");
} else {
Serial.println("Error reading humidity.");
}
delay(2000); // Wait for 2 seconds before the next reading
}
Connect your ESP32 to your computer via a USB cable, Ensure the correct Board and Port are selected under Tools, Click the "Upload" button in the Arduino IDE to compile and upload the code to your ESP32.
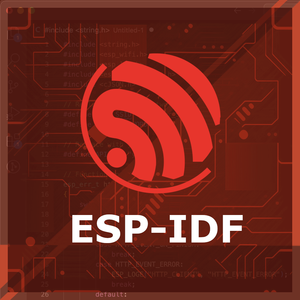
ESP32 HTE501 ESP-IDF Code ExampleExample in Espressif IoT Framework (ESP-IDF)
If you're using ESP-IDF to work with the HTE501 Temperature and Humidity Sensor, here's how you can set it up and read data from the sensor. Fill in this code in the main
ESP-IDF file:
#include <stdio.h>
#include "driver/i2c.h"
#include "esp_log.h"
#include "sdkconfig.h"
#define I2C_MASTER_SCL_IO 22 // GPIO number for I2C SCL
#define I2C_MASTER_SDA_IO 21 // GPIO number for I2C SDA
#define I2C_MASTER_NUM I2C_NUM_0 // I2C port number for master
#define I2C_MASTER_FREQ_HZ 100000 // I2C master clock frequency
#define I2C_MASTER_TX_BUF_DISABLE 0 // I2C master doesn't need buffer
#define I2C_MASTER_RX_BUF_DISABLE 0 // I2C master doesn't need buffer
#define HTE501_SENSOR_ADDR 0x44 // I2C address for the HTE501 sensor
#define HTE501_CMD_MEASURE 0x2C06 // Command for measurement
static const char *TAG = "HTE501";
void i2c_master_init() {
i2c_config_t conf = {
.mode = I2C_MODE_MASTER,
.sda_io_num = I2C_MASTER_SDA_IO,
.sda_pullup_en = GPIO_PULLUP_ENABLE,
.scl_io_num = I2C_MASTER_SCL_IO,
.scl_pullup_en = GPIO_PULLUP_ENABLE,
.master.clk_speed = I2C_MASTER_FREQ_HZ,
};
i2c_param_config(I2C_MASTER_NUM, &conf);
i2c_driver_install(I2C_MASTER_NUM, conf.mode, I2C_MASTER_RX_BUF_DISABLE, I2C_MASTER_TX_BUF_DISABLE, 0);
}
float read_humidity_temperature(float *temperature, float *humidity) {
uint8_t data[6];
i2c_cmd_handle_t cmd = i2c_cmd_link_create();
i2c_master_start(cmd);
i2c_master_write_byte(cmd, (HTE501_SENSOR_ADDR << 1) | I2C_MASTER_WRITE, true);
i2c_master_write_byte(cmd, HTE501_CMD_MEASURE >> 8, true);
i2c_master_write_byte(cmd, HTE501_CMD_MEASURE & 0xFF, true);
i2c_master_stop(cmd);
i2c_master_cmd_begin(I2C_MASTER_NUM, cmd, 1000 / portTICK_RATE_MS);
i2c_cmd_link_delete(cmd);
vTaskDelay(50 / portTICK_RATE_MS); // Wait for measurement
cmd = i2c_cmd_link_create();
i2c_master_start(cmd);
i2c_master_write_byte(cmd, (HTE501_SENSOR_ADDR << 1) | I2C_MASTER_READ, true);
i2c_master_read(cmd, data, 6, I2C_MASTER_LAST_NACK);
i2c_master_stop(cmd);
i2c_master_cmd_begin(I2C_MASTER_NUM, cmd, 1000 / portTICK_RATE_MS);
i2c_cmd_link_delete(cmd);
// Convert the data
uint16_t raw_temp = (data[0] << 8) | data[1];
uint16_t raw_hum = (data[3] << 8) | data[4];
*temperature = -45 + 175 * ((float)raw_temp / 65535.0);
*humidity = 100 * ((float)raw_hum / 65535.0);
return ESP_OK;
}
void app_main() {
i2c_master_init();
float temperature, humidity;
while (1) {
if (read_humidity_temperature(&temperature, &humidity) == ESP_OK) {
ESP_LOGI(TAG, "Temperature: %.2f °C", temperature);
ESP_LOGI(TAG, "Humidity: %.2f %%", humidity);
} else {
ESP_LOGE(TAG, "Could not read from sensor");
}
vTaskDelay(2000 / portTICK_RATE_MS); // Delay for 2 seconds
}
}
```<p>
Update the I2C pins (<code>I2C_MASTER_SDA_IO</code> and <code>I2C_MASTER_SCL_IO</code>) to match your ESP32 hardware setup, Use idf.py build to compile the project, Use idf.py flash to upload the code to your ESP32.
</p></div>
<!-- ESPHome Code -->
<div class="mb-5">
<div class="row d-flex">
<div class="col-12 col-lg-2 col-xl-1 text-center mb-2">
<picture><source type="image/avif" srcset="/img/1pBoXxHlpf-300.avif 300w"><source type="image/webp" srcset="/img/1pBoXxHlpf-300.webp 300w"><img alt="ESPHome Image" class="rounded-3" style="width: 100px; height: auto;" loading="lazy" decoding="async" src="/img/1pBoXxHlpf-300.png" width="300" height="300"></picture>
</div>
<div class="col-12 col-lg-10 col-xl-11 m-auto ps-3">
<h3 class="mb-1" id="hte501-esphome">ESP32 HTE501 ESPHome Code Example</h3>
<div style="font-size: 0.9rem;" class="text-secondary">Example in ESPHome (Home Assistant)</div>
</div>
</div>
<p>
Fill in this configuration in your ESPHome YAML configuration file (<code>example.yml</code>) to integrate the HTE501 Temperature and Humidity Sensor
</p>
```yaml
sensor:
- platform: hte501
temperature:
name: "Office Temperature"
humidity:
name: "Office Humidity"
address: 0x40
update_interval: 60s
Upload this code to your ESP32 using the ESPHome dashboard or the esphome run
command.
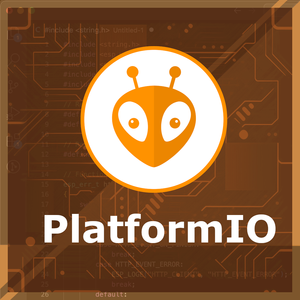
ESP32 HTE501 PlatformIO Code Example
For PlatformIO, make sure to configure the platformio.ini
file with the appropriate environment and libraries, and then proceed with the code.
Configure platformio.ini
First, your platformio.ini
should look like below. You might need to include some libraries as shown. Make sure to change the board to your ESP32:
[env:esp32dev]
platform = espressif32
board = esp32dev
framework = espidf
monitor_speed = 115200
lib_deps =
esp-idf-esp32
Wire
ESP32 HTE501 PlatformIO Example Code
Write this code in your PlatformIO project under the src/main.cpp
file to use the HTE501 Temperature and Humidity Sensor:
#include <stdio.h>
#include "driver/i2c.h"
#include "esp_log.h"
#define I2C_MASTER_SCL_IO 22 // GPIO number for I2C SCL
#define I2C_MASTER_SDA_IO 21 // GPIO number for I2C SDA
#define I2C_MASTER_NUM I2C_NUM_0 // I2C port number for master
#define I2C_MASTER_FREQ_HZ 100000 // I2C master clock frequency
#define I2C_MASTER_TX_BUF_DISABLE 0 // I2C master doesn't need buffer
#define I2C_MASTER_RX_BUF_DISABLE 0 // I2C master doesn't need buffer
#define HTE501_SENSOR_ADDR 0x44 // I2C address for the HTE501 sensor
#define HTE501_CMD_MEASURE 0x2C06 // Command for measurement
static const char *TAG = "HTE501";
void i2c_master_init() {
i2c_config_t conf = {
.mode = I2C_MODE_MASTER,
.sda_io_num = I2C_MASTER_SDA_IO,
.sda_pullup_en = GPIO_PULLUP_ENABLE,
.scl_io_num = I2C_MASTER_SCL_IO,
.scl_pullup_en = GPIO_PULLUP_ENABLE,
.master.clk_speed = I2C_MASTER_FREQ_HZ,
};
i2c_param_config(I2C_MASTER_NUM, &conf);
i2c_driver_install(I2C_MASTER_NUM, conf.mode, I2C_MASTER_RX_BUF_DISABLE, I2C_MASTER_TX_BUF_DISABLE, 0);
}
float read_humidity_temperature(float *temperature, float *humidity) {
uint8_t data[6];
i2c_cmd_handle_t cmd = i2c_cmd_link_create();
i2c_master_start(cmd);
i2c_master_write_byte(cmd, (HTE501_SENSOR_ADDR << 1) | I2C_MASTER_WRITE, true);
i2c_master_write_byte(cmd, HTE501_CMD_MEASURE >> 8, true);
i2c_master_write_byte(cmd, HTE501_CMD_MEASURE & 0xFF, true);
i2c_master_stop(cmd);
i2c_master_cmd_begin(I2C_MASTER_NUM, cmd, 1000 / portTICK_RATE_MS);
i2c_cmd_link_delete(cmd);
vTaskDelay(50 / portTICK_RATE_MS); // Wait for measurement
cmd = i2c_cmd_link_create();
i2c_master_start(cmd);
i2c_master_write_byte(cmd, (HTE501_SENSOR_ADDR << 1) | I2C_MASTER_READ, true);
i2c_master_read(cmd, data, 6, I2C_MASTER_LAST_NACK);
i2c_master_stop(cmd);
i2c_master_cmd_begin(I2C_MASTER_NUM, cmd, 1000 / portTICK_RATE_MS);
i2c_cmd_link_delete(cmd);
// Convert the data
uint16_t raw_temp = (data[0] << 8) | data[1];
uint16_t raw_hum = (data[3] << 8) | data[4];
*temperature = -45 + 175 * ((float)raw_temp / 65535.0);
*humidity = 100 * ((float)raw_hum / 65535.0);
return ESP_OK;
}
void app_main() {
i2c_master_init();
float temperature, humidity;
while (1) {
if (read_humidity_temperature(&temperature, &humidity) == ESP_OK) {
ESP_LOGI(TAG, "Temperature: %.2f °C", temperature);
ESP_LOGI(TAG, "Humidity: %.2f %%", humidity);
} else {
ESP_LOGE(TAG, "Could not read from sensor");
}
vTaskDelay(2000 / portTICK_RATE_MS); // Delay for 2 seconds
}
}
```<p>
Upload the code to your ESP32 using the PlatformIO "Upload" button in your IDE or the <code>pio run --target upload</code> command.
</p></div>
<!-- MicroPython Code -->
</div>
<h2>Conclusion</h2>
<p class="pb-5">
We went through technical specifications of HTE501 Temperature and Humidity Sensor, its pinout, connection with ESP32 and HTE501 Temperature and Humidity Sensor code examples with Arduino IDE, ESP-IDF, ESPHome and PlatformIO.
</p><div class="mb-5">
<h2>Similar <span class="text-capitalize">environment</span> Sensors</h2>
<div class="row d-flex"><div class="col-3 d-flex">
<a href="/sensors/sht30/" class="p-4 border rounded-3 text-decoration-none">
<div class="text-center h-75">
<picture><source type="image/avif" srcset="/img/r4CeFyArAR-200.avif 200w"><source type="image/webp" srcset="/img/r4CeFyArAR-200.webp 200w"><img alt="SHT30 Temperature and Humidity Sensor image" class="rounded-3" style="width: 200px; height: auto;" loading="lazy" decoding="async" src="/img/r4CeFyArAR-200.png" width="200" height="200"></picture>
</div>
<div class="mt-4 text-center">
<strong>SHT30 Temperature and Humidity Sensor</strong>
</div>
</a>
</div><div class="col-3 d-flex">
<a href="/sensors/bmp085/" class="p-4 border rounded-3 text-decoration-none">
<div class="text-center h-75">
<picture><source type="image/avif" srcset="/img/LgMeai43VW-200.avif 200w"><source type="image/webp" srcset="/img/LgMeai43VW-200.webp 200w"><img alt="BMP085 Barometric Pressure Sensor image" class="rounded-3" style="width: 200px; height: auto;" loading="lazy" decoding="async" src="/img/LgMeai43VW-200.png" width="200" height="200"></picture>
</div>
<div class="mt-4 text-center">
<strong>BMP085 Barometric Pressure Sensor</strong>
</div>
</a>
</div><div class="col-3 d-flex">
<a href="/sensors/bme280/" class="p-4 border rounded-3 text-decoration-none">
<div class="text-center h-75">
<picture><source type="image/avif" srcset="/img/y02He3uZkO-200.avif 200w"><source type="image/webp" srcset="/img/y02He3uZkO-200.webp 200w"><img alt="BME280 Temperature and Humidity Sensor image" class="rounded-3" style="width: 200px; height: auto;" loading="lazy" decoding="async" src="/img/y02He3uZkO-200.png" width="200" height="199"></picture>
</div>
<div class="mt-4 text-center">
<strong>BME280 Temperature and Humidity Sensor</strong>
</div>
</a>
</div><div class="col-3 d-flex">
<a href="/sensors/sht21/" class="p-4 border rounded-3 text-decoration-none">
<div class="text-center h-75">
<picture><source type="image/avif" srcset="/img/PEg2GoyNmv-200.avif 200w"><source type="image/webp" srcset="/img/PEg2GoyNmv-200.webp 200w"><img alt="SHT21 / HTU21 / GY-21 / SI7021 Temperature and Humidity Sensor image" class="rounded-3" style="width: 200px; height: auto;" loading="lazy" decoding="async" src="/img/PEg2GoyNmv-200.png" width="200" height="200"></picture>
</div>
<div class="mt-4 text-center">
<strong>SHT21 / HTU21 / GY-21 / SI7021 Temperature and Humidity Sensor</strong>
</div>
</a>
</div></div>
</div></div>