ESP32 JSN-SR04T Waterproof Ultrasonic Distance Sensor
The JSN-SR04T is a waterproof ultrasonic distance sensor ideal for outdoor and industrial applications. With a measuring range of up to 6 meters and a durable build, it is perfect for detecting objects in harsh conditions. It operates using Trigger and Echo signals and is compatible with microcontrollers like Arduino and ESP32.
Jump to Code Examples
Quick Links
JSN-SR04T Price
About JSN-SR04T Waterproof Ultrasonic Distance Sensor
The JSN-SR04T is a waterproof ultrasonic distance sensor capable of measuring distances from 25 cm to 600 cm. Its robust design makes it suitable for outdoor and industrial applications, such as liquid level measurement, parking sensors, and proximity detection. The sensor operates using Trigger and Echo pins, similar to the HC-SR04, and works well in environments where water or dust exposure is a concern.JSN-SR04T Sensor Technical Specifications
Below you can see the JSN-SR04T Waterproof Ultrasonic Distance Sensor Technical Specifications. The sensor is compatible with the ESP32, operating within a voltage range suitable for microcontrollers. For precise details about its features, specifications, and usage, refer to the sensor’s datasheet.
- Protocol: Trigger/Echo
- Measurement Range: 25 cm to 600 cm
- Resolution: 0.5 cm
- Accuracy: ±1 cm
- Operating Voltage: 5V DC
- Trigger Pulse Duration: 10 µs
- Interface: Trigger/Echo
JSN-SR04T Sensor Pinout
Below you can see the pinout for the JSN-SR04T Waterproof Ultrasonic Distance Sensor. The VCC
pin is used to supply power to the sensor, and it typically requires 3.3V or 5V (refer to the datasheet for specific voltage requirements). The GND
pin is the ground connection and must be connected to the ground of your ESP32!
The JSN-SR04T pinout is as follows:
- VCC: Connect to a 5V power supply.
- GND: Ground connection.
- Trigger (TRIG): Input pin to initiate distance measurement by sending a short 10 µs pulse.
- Echo: Output pin that provides a pulse width corresponding to the measured distance.
JSN-SR04T Wiring with ESP32
Below you can see the wiring for the JSN-SR04T Waterproof Ultrasonic Distance Sensor with the ESP32. Connect the VCC pin of the sensor to the 3.3V pin on the ESP32 or external power supply for power and the GND pin of the sensor to the GND pin of the ESP32. Depending on the communication protocol of the sensor (e.g., I2C, SPI, UART, or analog), connect the appropriate data and clock or signal pins to compatible GPIO pins on the ESP32, as shown below in the wiring diagram.
VCC
to a 5V power source, GND
to ground, and the Trigger
and Echo
pins to two GPIO pins on the microcontroller. Ensure proper resistors or level shifters are used if interfacing with 3.3V logic devices.Code Examples
Below you can find code examples of JSN-SR04T Waterproof Ultrasonic Distance Sensor with ESP32 in several frameworks:
If you encounter issues while using the JSN-SR04T Waterproof Ultrasonic Distance Sensor, check the Common Issues Troubleshooting Guide.
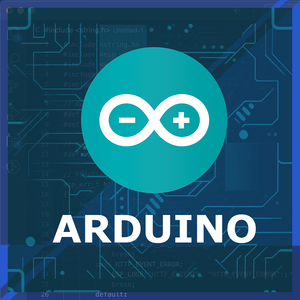
ESP32 JSN-SR04T Arduino IDE Code Example
Fill in your main
Arduino IDE sketch file with the following code to use the JSN-SR04T Waterproof Ultrasonic Distance Sensor:
#define TRIG_PIN 5
#define ECHO_PIN 18
void setup() {
Serial.begin(115200);
pinMode(TRIG_PIN, OUTPUT);
pinMode(ECHO_PIN, INPUT);
Serial.println("JSN-SR04T Distance Sensor Example");
}
void loop() {
long duration;
float distance;
// Trigger the sensor
digitalWrite(TRIG_PIN, LOW);
delayMicroseconds(2);
digitalWrite(TRIG_PIN, HIGH);
delayMicroseconds(10);
digitalWrite(TRIG_PIN, LOW);
// Read the Echo pin
duration = pulseIn(ECHO_PIN, HIGH);
// Calculate distance in cm
distance = duration * 0.034 / 2;
Serial.print("Distance: ");
Serial.print(distance);
Serial.println(" cm");
delay(500);
}
This Arduino sketch demonstrates how to use the JSN-SR04T sensor for distance measurement. The TRIG_PIN
and ECHO_PIN
are defined to connect the sensor to GPIO pins 9 and 10, respectively. The setup()
function initializes these pins and configures the Serial Monitor. In the loop()
, a 10 µs pulse is sent to the Trigger pin to start measurement, and the duration of the Echo pin's HIGH state is measured using the pulseIn()
function. The distance is calculated using the formula duration * 0.034 / 2
, which converts the time into distance in centimeters.
Connect your ESP32 to your computer via a USB cable, Ensure the correct Board and Port are selected under Tools, Click the "Upload" button in the Arduino IDE to compile and upload the code to your ESP32.
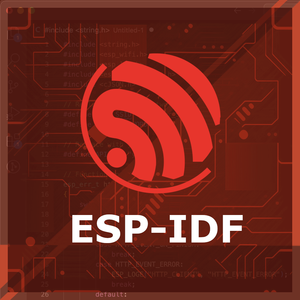
ESP32 JSN-SR04T ESP-IDF Code ExampleExample in Espressif IoT Framework (ESP-IDF)
If you're using ESP-IDF to work with the JSN-SR04T Waterproof Ultrasonic Distance Sensor, here's how you can set it up and read data from the sensor. Fill in this code in the main
ESP-IDF file:
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "freertos/task.h"
#include "driver/gpio.h"
#include "esp_timer.h"
#define TRIG_PIN GPIO_NUM_5
#define ECHO_PIN GPIO_NUM_18
void app_main() {
gpio_set_direction(TRIG_PIN, GPIO_MODE_OUTPUT);
gpio_set_direction(ECHO_PIN, GPIO_MODE_INPUT);
while (1) {
// Send trigger pulse
gpio_set_level(TRIG_PIN, 0);
ets_delay_us(2);
gpio_set_level(TRIG_PIN, 1);
ets_delay_us(10);
gpio_set_level(TRIG_PIN, 0);
// Measure echo pulse width
uint64_t start_time = esp_timer_get_time();
while (!gpio_get_level(ECHO_PIN)); // Wait for HIGH
uint64_t echo_start = esp_timer_get_time();
while (gpio_get_level(ECHO_PIN)); // Wait for LOW
uint64_t echo_end = esp_timer_get_time();
uint64_t duration = echo_end - echo_start;
float distance = (duration * 0.034) / 2;
printf("Distance: %.2f cm\n", distance);
vTaskDelay(pdMS_TO_TICKS(500));
}
}
This ESP-IDF code configures GPIO pins for the Trigger and Echo of the JSN-SR04T sensor. The gpio_set_level()
function sends a 10 µs pulse to the Trigger pin. The time taken for the Echo pin to go HIGH and then LOW is measured using esp_timer_get_time()
, which provides timestamps in microseconds. The distance is calculated based on the formula (duration * 0.034) / 2
. The program continuously measures and prints the distance in centimeters every 500 ms.
Update the I2C pins (I2C_MASTER_SDA_IO
and I2C_MASTER_SCL_IO
) to match your ESP32 hardware setup, Use idf.py build to compile the project, Use idf.py flash to upload the code to your ESP32.
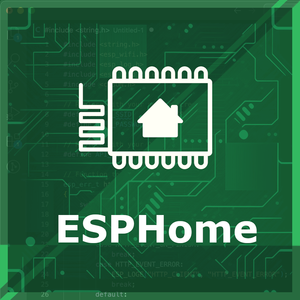
ESP32 JSN-SR04T ESPHome Code Example
Fill in this configuration in your ESPHome YAML configuration file (example.yml
) to integrate the JSN-SR04T Waterproof Ultrasonic Distance Sensor
sensor:
- platform: ultrasonic
trigger_pin: GPIO5
echo_pin: GPIO18
name: "JSN-SR04T Distance"
update_interval: 500ms
accuracy_decimals: 1
timeout: 2.0m
The ESPHome configuration uses the ultrasonic
platform to interface with the JSN-SR04T sensor. The trigger_pin
and echo_pin
specify the GPIO pins connected to the sensor. The name
assigns a user-friendly identifier ('JSN-SR04T Distance') for use in platforms like Home Assistant. The update_interval
of 500 ms specifies how often distance measurements are taken, while accuracy_decimals
ensures measurements are displayed to one decimal place. The timeout
prevents errors in case of no response within the specified time.
Upload this code to your ESP32 using the ESPHome dashboard or the esphome run
command.
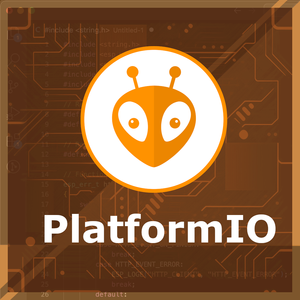
ESP32 JSN-SR04T PlatformIO Code Example
For PlatformIO, make sure to configure the platformio.ini
file with the appropriate environment and libraries, and then proceed with the code.
Configure platformio.ini
First, your platformio.ini
should look like below. You might need to include some libraries as shown. Make sure to change the board to your ESP32:
[env:esp32dev]
platform = espressif32
board = esp32dev
framework = arduino
monitor_speed = 115200
ESP32 JSN-SR04T PlatformIO Example Code
Write this code in your PlatformIO project under the src/main.cpp
file to use the JSN-SR04T Waterproof Ultrasonic Distance Sensor:
#define TRIG_PIN 5
#define ECHO_PIN 18
void setup() {
Serial.begin(115200);
pinMode(TRIG_PIN, OUTPUT);
pinMode(ECHO_PIN, INPUT);
}
void loop() {
long duration;
float distance;
// Trigger the sensor
digitalWrite(TRIG_PIN, LOW);
delayMicroseconds(2);
digitalWrite(TRIG_PIN, HIGH);
delayMicroseconds(10);
digitalWrite(TRIG_PIN, LOW);
// Read the Echo pin
duration = pulseIn(ECHO_PIN, HIGH);
// Calculate distance
distance = duration * 0.034 / 2;
Serial.print("Distance: ");
Serial.print(distance);
Serial.println(" cm");
delay(500);
}
The PlatformIO code is identical to the Arduino example, making it compatible with ESP32 boards configured via the PlatformIO environment. It uses TRIG_PIN
and ECHO_PIN
for sensor operation. A short 10 µs pulse triggers the measurement, and the pulse duration is read on the Echo pin using the pulseIn()
function. The calculated distance is printed to the Serial Monitor every 500 ms.
Upload the code to your ESP32 using the PlatformIO "Upload" button in your IDE or the pio run --target upload
command.
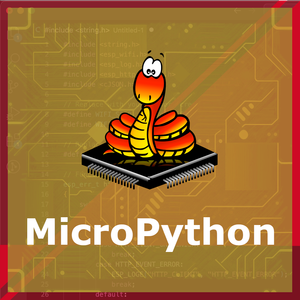
ESP32 JSN-SR04T MicroPython Code Example
Fill in this script in your MicroPython main.py file (main.py
) to integrate the JSN-SR04T Waterproof Ultrasonic Distance Sensor with your ESP32.
from machine import Pin, time_pulse_us
from time import sleep
# Define pins for Trigger and Echo
TRIG_PIN = 5
ECHO_PIN = 18
# Initialize Trigger and Echo pins
trig = Pin(TRIG_PIN, Pin.OUT)
echo = Pin(ECHO_PIN, Pin.IN)
def measure_distance():
# Send a 10 µs pulse to the Trigger pin
trig.low()
sleep(0.000002) # 2 µs
trig.high()
sleep(0.00001) # 10 µs
trig.low()
# Measure the duration of the Echo pulse
duration = time_pulse_us(echo, 1, 30000) # Timeout after 30 ms (no response)
# Calculate distance in cm (speed of sound = 343 m/s)
distance = (duration * 0.0343) / 2
return distance
print("JSN-SR04T Distance Sensor Example")
while True:
distance = measure_distance()
if distance > 0:
print("Distance: {:.2f} cm".format(distance))
else:
print("Out of range or no object detected.")
sleep(0.5)
This MicroPython script interfaces with the JSN-SR04T sensor using Trigger and Echo pins. The Trigger pin sends a 10 µs pulse to initiate the measurement, while the Echo pin receives a pulse whose width corresponds to the distance measured. The time_pulse_us()
function measures the duration of the Echo pulse in microseconds, with a timeout of 30 ms to prevent infinite waiting. The distance is calculated using the formula (duration * 0.0343) / 2
, where 0.0343 cm/µs is the speed of sound. The script continuously measures and prints the distance to the console every 500 ms. If no response is detected, it prints an 'Out of range' message.
Upload this code to your ESP32 using a MicroPython-compatible IDE, such as Thonny, uPyCraft, or tools like ampy
.
JSN-SR04T Waterproof Ultrasonic Distance Sensor Troubleshooting
This guide outlines a systematic approach to troubleshoot and resolve common problems with the . Start by confirming that the hardware connections are correct, as wiring mistakes are the most frequent cause of issues. If you are sure the connections are correct, follow the below steps to debug common issues.
Sensor Returns Constant or Erroneous Readings
Issue: The JSN-SR04T sensor provides constant or incorrect distance measurements, regardless of the actual distance to the target.
Possible causes include incorrect wiring, insufficient power supply, or improper sensor configuration.
Solution: Ensure that the sensor is connected to a stable 5V power source, as it requires 5V for proper operation. Verify that the TRIG and ECHO pins are correctly connected to the appropriate GPIO pins on the microcontroller. Confirm that the sensor is properly initialized in your code, and that the trigger pulse duration is set correctly; some users have found that a 15-microsecond trigger pulse can improve reliability.
Inconsistent or Fluctuating Distance Measurements
Issue: The sensor outputs distance readings that vary significantly, even when the target distance remains constant.
Possible causes include environmental factors such as temperature variations, soft or angled target surfaces, or electrical noise.
Solution: Position the sensor perpendicular to a hard, flat target surface to ensure accurate reflections. Be aware that temperature changes can affect the speed of sound; consider implementing temperature compensation if precise measurements are required. Implement averaging of multiple readings in your code to mitigate occasional erroneous data.
Sensor Not Detected or Unresponsive
Issue: The microcontroller fails to detect the JSN-SR04T sensor, or the sensor does not respond to trigger signals.
Possible causes include incorrect pin assignments in the code, lack of proper initialization, or defective sensor module.
Solution: Double-check the pin assignments in your code to ensure they match the physical connections. Confirm that the sensor is properly initialized in the setup section of your code. If the issue persists, test the sensor with a known working setup or replace it to rule out hardware failure.
Interference from Environmental Factors
Issue: External factors cause the sensor to produce unreliable readings.
Possible causes include high ambient noise levels, temperature variations, or obstacles in the sensor's field of view.
Solution: Operate the sensor in a controlled environment to minimize acoustic and electrical noise. Ensure that there are no unintended obstacles within the sensor's detection range that could cause false readings.
Conclusion
We went through technical specifications of JSN-SR04T Waterproof Ultrasonic Distance Sensor, its pinout, connection with ESP32 and JSN-SR04T Waterproof Ultrasonic Distance Sensor code examples with Arduino IDE, ESP-IDF, ESPHome and PlatformIO.