ESP32 SIM800C GSM/GPRS Module
The SIM800C is a versatile GSM/GPRS module that provides quad-band connectivity for voice, SMS, and data applications. Its compact design and low power requirements make it suitable for a wide range of communication projects.
π Quick Links
π SIM800C Price
βΉοΈ About SIM800C GSM/GPRS Module
The SIM800C is a quad-band GSM/GPRS module designed for voice, SMS, and data transmission. With its compact size and low power consumption, it is ideal for wearables, IoT devices, and industrial automation.
β‘ Key Features #
- Quad-Band GSM (850/900/1800/1900MHz) β Ensures global network compatibility.
- Versatile Communication β Supports voice calls, SMS, and GPRS data.
- Multiple Interfaces β Includes UART and USB for flexible integration.
- Additional Features β Supports Bluetooth and FM, enhancing its functionality.
π Still deciding on a SIM module? Check the ESP32 SIM Modules Comparison Table for a breakdown of LTE, 3G, and GPRS options. π
βοΈ SIM800C Sensor Technical Specifications
Below you can see the SIM800C GSM/GPRS Module Technical Specifications. The sensor is compatible with the ESP32, operating within a voltage range suitable for microcontrollers. For precise details about its features, specifications, and usage, refer to the sensorβs datasheet.
- Type: sim
- Protocol: UART
- Frequency Bands: Quad-band 850/900/1800/1900MHz
- Supply Voltage: 3.4V to 4.4V
- Power Consumption (Sleep Mode): 0.8mA
- Dimensions: 17.6mm x 15.7mm x 2.3mm
- Operating Temperature: -40Β°C to +85Β°C
- GPRS Connectivity: GPRS multi-slot class 12
- SIM Card Support: 1.8V and 3V SIM cards
- Interfaces: UART, USB, SIM, GPIO
π SIM800C Sensor Pinout
Below you can see the pinout for the SIM800C GSM/GPRS Module. The VCC
pin is used to supply power to the sensor, and it typically requires 3.3V or 5V (refer to the datasheet for specific voltage requirements). The GND
pin is the ground connection and must be connected to the ground of your ESP32!
The SIM800C pinout includes:
- VBAT: Power supply input (3.4V to 4.4V).
- GND: Ground connection.
- UART1_TXD: UART Transmit Data (connects to microcontroller RX).
- UART1_RXD: UART Receive Data (connects to microcontroller TX).
- PWRKEY: Power on/off control (active low).
- NETLIGHT: Network status indication.
- STATUS: Module operating status indication.
- SIM_VDD: SIM card power supply.
- SIM_DATA: SIM card data I/O.
- SIM_CLK: SIM card clock.
- SIM_RST: SIM card reset.
π§΅ SIM800C Wiring with ESP32
Below you can see the wiring for the SIM800C GSM/GPRS Module with the ESP32. Connect the VCC pin of the sensor to the 3.3V pin on the ESP32 or external power supply for power and the GND pin of the sensor to the GND pin of the ESP32. Depending on the communication protocol of the sensor (e.g., I2C, SPI, UART, or analog), connect the appropriate data and clock or signal pins to compatible GPIO pins on the ESP32, as shown below in the wiring diagram.
- Connect
VBAT
to a stable power supply within the range of 3.4V to 4.4V. - Connect
GND
to the ground of the microcontroller. - Connect
UART1_TXD
to the RX pin of the microcontroller andUART1_RXD
to the TX pin. - Connect
PWRKEY
to a GPIO pin on the microcontroller; to power on the module, pull this pin low for at least 1 second. - Optionally, connect
NETLIGHT
andSTATUS
pins to LEDs for network and status indications. - For SIM card connections, connect
SIM_VDD
,SIM_DATA
,SIM_CLK
, andSIM_RST
to the corresponding pins on the SIM card holder.
π οΈ SIM800C GSM/GPRS Module Troubleshooting
This guide outlines a systematic approach to troubleshoot and resolve common problems with the . Start by confirming that the hardware connections are correct, as wiring mistakes are the most frequent cause of issues. If you are sure the connections are correct, follow the below steps to debug common issues.
β Module Fails to Power On
Issue: The SIM800C module does not power up or respond to commands.
Possible causes include insufficient power supply, incorrect wiring, or faulty hardware.
Solution: Ensure the module is connected to a stable power source within the recommended voltage range of 3.4V to 4.4V. Verify that all connections are secure and correctly configured. If the problem persists, consider testing the module with a different power source or replacing it.
πΆ SIM Card Not Recognized
Issue: The module fails to detect or register the SIM card.
Possible causes include improper SIM card insertion, unsupported SIM card type, or SIM card lock.
Solution: Ensure the SIM card is properly inserted into the module's SIM card slot and is compatible with the GSM network. Verify that the SIM card is active and unlocked. If necessary, test the SIM card in another device to confirm its functionality.
β οΈ Poor Network Signal or Connectivity Issues
Issue: The module experiences weak signal strength or fails to maintain a stable network connection.
Possible causes include improper antenna connection, environmental interference, or network coverage limitations.
Solution: Ensure the GSM antenna is securely connected to the module and positioned for optimal signal reception. Avoid placing the module near sources of electromagnetic interference. Check the network coverage in your area to ensure adequate signal strength.
π¬ AT Commands Not Responding
Issue: The module does not respond to AT commands sent from the microcontroller or computer.
Possible causes include incorrect baud rate settings, faulty serial connections, or improper command syntax.
Solution: Verify that the baud rate of the module matches that of the microcontroller or computer; the default baud rate is 9600 bps. Check that the TX and RX lines are correctly connected and that there are no loose connections. Ensure that AT commands are correctly formatted and terminated with a carriage return.
π₯ Module Overheating
Issue: The SIM800C module becomes excessively hot during operation.
Possible causes include overvoltage, excessive current draw, or continuous high-power transmission.
Solution: Confirm that the power supply voltage is within the recommended range (3.4V to 4.4V). Monitor the current consumption to ensure it does not exceed the module's specifications. If the module is transmitting continuously, consider implementing power-saving modes or reducing the transmission frequency to prevent overheating.
π» Code Examples
Below you can find code examples of SIM800C GSM/GPRS Module with ESP32 in several frameworks:
If you encounter issues while using the SIM800C GSM/GPRS Module, check the Common Issues Troubleshooting Guide.
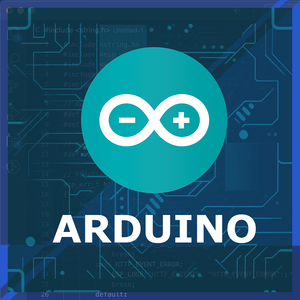
ESP32 SIM800C Arduino IDE Code Example
Fill in your main
Arduino IDE sketch file with the following code to use the SIM800C GSM/GPRS Module:
#include <SoftwareSerial.h>
SoftwareSerial sim800c(10, 11); // RX, TX
void setup() {
Serial.begin(9600);
sim800c.begin(9600);
// Power on the module
pinMode(9, OUTPUT);
digitalWrite(9, LOW);
delay(1000); // PWRKEY needs to be low for at least 1 second
digitalWrite(9, HIGH);
delay(5000); // Wait for the module to initialize
// Test AT communication
sim800c.println("AT");
delay(1000);
while (sim800c.available()) {
Serial.write(sim800c.read());
}
}
void loop() {
// Send an SMS
sim800c.println("AT+CMGF=1"); // Set SMS to text mode
delay(1000);
sim800c.println("AT+CMGS=\"+1234567890\""); // Replace with recipient's number
delay(1000);
sim800c.print("Hello from SIM800C");
delay(1000);
sim800c.write(26); // CTRL+Z to send SMS
delay(5000);
}
This Arduino sketch interfaces with the SIM800C module using the SoftwareSerial library. The module is powered on by toggling the PWRKEY pin. An AT command is sent to verify communication. In the loop, the sketch sends an SMS by setting the module to SMS text mode, specifying the recipient's number, and sending the message, finalized with CTRL+Z (ASCII 26) to initiate transmission.
Connect your ESP32 to your computer via a USB cable, Ensure the correct Board and Port are selected under Tools, Click the "Upload" button in the Arduino IDE to compile and upload the code to your ESP32.
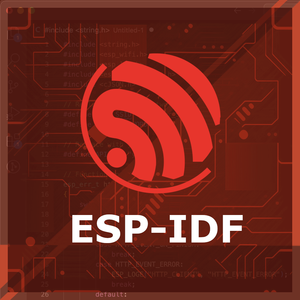
ESP32 SIM800C ESP-IDF Code ExampleExample in Espressif IoT Framework (ESP-IDF)
If you're using ESP-IDF to work with the SIM800C GSM/GPRS Module, here's how you can set it up and read data from the sensor. Fill in this code in the main
ESP-IDF file:
#include <stdio.h>
#include "driver/uart.h"
#include "driver/gpio.h"
#include "freertos/task.h"
#define TX_PIN 17
#define RX_PIN 16
#define PWRKEY_PIN 4
#define UART_PORT UART_NUM_1
void init_uart() {
uart_config_t uart_config = {
.baud_rate = 9600,
.data_bits = UART_DATA_8_BITS,
.parity = UART_PARITY_DISABLE,
.stop_bits = UART_STOP_BITS_1,
.flow_ctrl = UART_HW_FLOWCTRL_DISABLE
};
uart_param_config(UART_PORT, &uart_config);
uart_set_pin(UART_PORT, TX_PIN, RX_PIN, UART_PIN_NO_CHANGE, UART_PIN_NO_CHANGE);
uart_driver_install(UART_PORT, 1024, 0, 0, NULL, 0);
}
void power_on_sim800c() {
gpio_set_direction(PWRKEY_PIN, GPIO_MODE_OUTPUT);
gpio_set_level(PWRKEY_PIN, 0);
vTaskDelay(1000 / portTICK_PERIOD_MS); // Hold PWRKEY low for 1 second
gpio_set_level(PWRKEY_PIN, 1);
vTaskDelay(5000 / portTICK_PERIOD_MS); // Wait for the module to initialize
}
void app_main(void) {
init_uart();
power_on_sim800c();
char *test_cmd = "AT\r\n";
uart_write_bytes(UART_PORT, test_cmd, strlen(test_cmd));
while (true) {
char data[128];
int len = uart_read_bytes(UART_PORT, data, sizeof(data), 100 / portTICK_PERIOD_MS);
if (len > 0) {
data[len] = '\0';
printf("Response: %s\n", data);
}
vTaskDelay(1000 / portTICK_PERIOD_MS);
}
}
This ESP-IDF example initializes UART communication with the SIM800C module and powers it on using the PWRKEY pin (GPIO4). The UART interface is configured with GPIO17 as TX and GPIO16 as RX. An AT command is sent to test communication, and responses from the module are printed to the console. The function `power_on_sim800c()` toggles the PWRKEY pin to activate the module.
Update the I2C pins (I2C_MASTER_SDA_IO
and I2C_MASTER_SCL_IO
) to match your ESP32 hardware setup, Use idf.py build to compile the project, Use idf.py flash to upload the code to your ESP32.
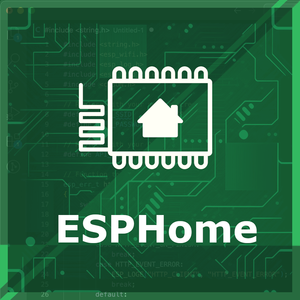
ESP32 SIM800C ESPHome Code Example
Fill in this configuration in your ESPHome YAML configuration file (example.yml
) to integrate the SIM800C GSM/GPRS Module
uart:
tx_pin: GPIO17
rx_pin: GPIO16
baud_rate: 9600
switch:
- platform: gpio
name: "SIM800C Power"
pin:
number: GPIO4
inverted: true
switch:
- platform: template
name: "Send AT Command"
turn_on_action:
- uart.write: "AT\r\n"
sensor:
- platform: custom
lambda: |-
return {nullptr};
sensors:
- name: "SIM800C Response"
This ESPHome configuration sets up UART communication with the SIM800C using GPIO17 (TX) and GPIO16 (RX) at 9600 baud. A GPIO-based switch is used to control the PWRKEY pin (GPIO4) for powering the module on or off. A template switch allows sending the AT command, and a custom sensor can be implemented to process responses from the module.
Upload this code to your ESP32 using the ESPHome dashboard or the esphome run
command.
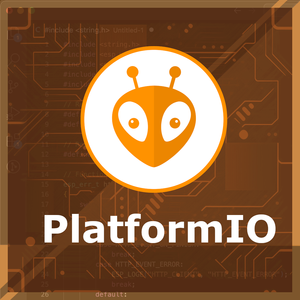
ESP32 SIM800C PlatformIO Code Example
For PlatformIO, make sure to configure the platformio.ini
file with the appropriate environment and libraries, and then proceed with the code.
Configure platformio.ini
First, your platformio.ini
should look like below. You might need to include some libraries as shown. Make sure to change the board to your ESP32:
[env:sim800c]
platform = espressif32
board = esp32dev
framework = arduino
monitor_speed = 115200
ESP32 SIM800C PlatformIO Example Code
Write this code in your PlatformIO project under the src/main.cpp
file to use the SIM800C GSM/GPRS Module:
#include <HardwareSerial.h>
#include <Arduino.h>
HardwareSerial sim800c(1);
#define PWRKEY 4
void power_on_sim800c() {
pinMode(PWRKEY, OUTPUT);
digitalWrite(PWRKEY, LOW);
delay(1000); // Hold PWRKEY low for 1 second
digitalWrite(PWRKEY, HIGH);
delay(5000); // Wait for initialization
}
void setup() {
Serial.begin(115200);
sim800c.begin(9600, SERIAL_8N1, 16, 17); // RX, TX
power_on_sim800c();
// Test AT command
sim800c.println("AT");
delay(1000);
while (sim800c.available()) {
Serial.write(sim800c.read());
}
}
void loop() {
sim800c.println("AT+CMGF=1"); // Set SMS to text mode
delay(1000);
sim800c.println("AT+CMGS=\"+1234567890\""); // Replace with recipient's number
delay(1000);
sim800c.print("Hello from PlatformIO");
delay(1000);
sim800c.write(26); // CTRL+Z to send SMS
delay(5000);
}
This PlatformIO code interfaces with the SIM800C module using HardwareSerial on an ESP32. The `power_on_sim800c` function toggles the PWRKEY pin (GPIO4) to activate the module. The AT command is sent to test communication, and SMS functionality is implemented in the loop. GPIO16 (RX) and GPIO17 (TX) are configured as serial pins.
Upload the code to your ESP32 using the PlatformIO "Upload" button in your IDE or the pio run --target upload
command.
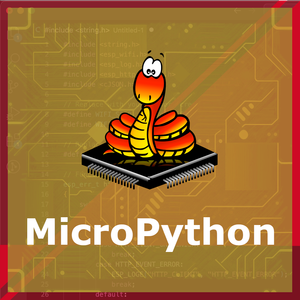
ESP32 SIM800C MicroPython Code Example
Fill in this script in your MicroPython main.py file (main.py
) to integrate the SIM800C GSM/GPRS Module with your ESP32.
from machine import UART, Pin
import time
# Initialize UART
uart = UART(2, baudrate=9600, tx=17, rx=16)
pwrkey = Pin(4, Pin.OUT)
def power_on_sim800c():
pwrkey.value(0)
time.sleep(1) # Hold PWRKEY low for 1 second
pwrkey.value(1)
time.sleep(5) # Wait for module to initialize
def send_at(command):
uart.write(command + '\r\n')
time.sleep(1)
while uart.any():
print(uart.read().decode('utf-8'), end='')
# Power on the module
power_on_sim800c()
# Test communication
send_at('AT')
# Send SMS
send_at('AT+CMGF=1') # Set SMS to text mode
send_at('AT+CMGS="+1234567890"') # Replace with recipient's number
uart.write("Hello from MicroPython" + chr(26))
This MicroPython code communicates with the SIM800C module over UART. The `power_on_sim800c` function activates the module using the PWRKEY pin (GPIO4). The `send_at` function sends AT commands and prints the responses. The script initializes the module, tests communication, and sends an SMS with a specified message.
Upload this code to your ESP32 using a MicroPython-compatible IDE, such as Thonny, uPyCraft, or tools like ampy
.
Conclusion
We went through technical specifications of SIM800C GSM/GPRS Module, its pinout, connection with ESP32 and SIM800C GSM/GPRS Module code examples with Arduino IDE, ESP-IDF, ESPHome and PlatformIO.