ESP32 AGS10 Sensor
The AGS10 is a gas sensor known for detecting a range of gases, including methane, propane, and hydrogen. Designed with stability and sensitivity, it’s suitable for industrial safety and environmental monitoring. The sensor offers a rapid response time, high sensitivity, and low power consumption, often used in applications like leak detection and air quality monitoring systems.
🔗 Quick Links
ℹ️ About AGS10 Sensor
The AGS10 is a compact and highly sensitive gas sensor designed for air quality monitoring and gas detection applications. It can detect various gases, including volatile organic compounds (VOCs), making it useful for indoor air monitoring, industrial safety, and smart home projects.
⚡ Key Features #
- High Sensitivity to VOCs – Ideal for air quality monitoring and gas detection.
- Compact & Low Power – Suitable for battery-powered applications.
- Fast Response & Recovery Time – Ensures real-time gas monitoring.
- I²C Communication – Simplifies integration with ESP32 and other microcontrollers.
⚙️ AGS10 Sensor Technical Specifications
Below you can see the AGS10 Sensor Technical Specifications. The sensor is compatible with the ESP32, operating within a voltage range suitable for microcontrollers. For precise details about its features, specifications, and usage, refer to the sensor’s datasheet.
- Type: airQuality
- Protocol: I2C
- Interface: I2C
- Measuring range: 0~99999 ppb
- Accuracy: 25% reading
- Operating Range: 0~50 ℃, 0~95%RH
- Voltage: 3.0 ± 0.1V DC
🔌 AGS10 Sensor Pinout
Below you can see the pinout for the AGS10 Sensor. The VCC
pin is used to supply power to the sensor, and it typically requires 3.3V or 5V (refer to the datasheet for specific voltage requirements). The GND
pin is the ground connection and must be connected to the ground of your ESP32!
🧵 AGS10 Wiring with ESP32
Below you can see the wiring for the AGS10 Sensor with the ESP32. Connect the VCC pin of the sensor to the 3.3V pin on the ESP32 or external power supply for power and the GND pin of the sensor to the GND pin of the ESP32. Depending on the communication protocol of the sensor (e.g., I2C, SPI, UART, or analog), connect the appropriate data and clock or signal pins to compatible GPIO pins on the ESP32, as shown below in the wiring diagram.
🛠️ AGS10 Sensor Troubleshooting
This guide outlines a systematic approach to troubleshoot and resolve common problems with the . Start by confirming that the hardware connections are correct, as wiring mistakes are the most frequent cause of issues. If you are sure the connections are correct, follow the below steps to debug common issues.
⚠️ CRC Error on ESP32-S2
Issue: When using the AGS10 sensor with an ESP32-S2 microcontroller, the following error is encountered: The crc check failed
. This issue arises despite the sensor functioning correctly on standard ESP32 boards.
Possible causes include the AGS10 sensor's requirement for an I2C bus speed not exceeding 15kHz, which may not be properly configured on the ESP32-S2.
Solution: Ensure that the I2C bus frequency is explicitly set to 15kHz in your configuration. In ESPHome, this can be achieved by specifying frequency: 15kHz
in the I2C setup. Additionally, verify that the ESP32-S2 supports the specified I2C frequency and that there are no hardware limitations affecting communication.
🔌 Connection Issues with TCA9548A Multiplexer
Issue: Integrating the AGS10 sensor with a TCA9548A I2C multiplexer in ESPHome results in configuration errors, such as: required key not provided
.
Possible causes include incorrect or incomplete configuration settings in the ESPHome YAML file, particularly when defining the multiplexer channels.
Solution: Review the ESPHome configuration to ensure that all required keys and parameters are correctly specified. Each multiplexer channel should be properly defined with the necessary settings. Consulting the ESPHome documentation for guidance on configuring I2C multiplexers can provide clarity.
💻 Compilation Errors with AGS10 Library
Issue: When compiling code that includes the AGS10 sensor library, errors such as: invalid conversion from 'uint8_t' {aka 'unsigned char'} to 'uint8_t*' {aka 'unsigned char*'}
occur.
Possible causes include incompatibilities in the AGS10 library when used with certain microcontrollers, such as boards other than the Arduino Uno.
Solution: Consider using a modified version of the AGS10 library that addresses these compatibility issues. For instance, a fork of the library tailored for ESP32 is available and may resolve the compilation errors.
🔧 Incorrect I2C Bus Frequency Configuration
Issue: The AGS10 sensor requires an I2C bus speed not exceeding 15kHz. Failure to configure this can lead to communication errors or sensor malfunction.
Possible causes include the default I2C bus frequency being set higher than the sensor's specifications.
Solution: Explicitly set the I2C bus frequency to 15kHz in your microcontroller's configuration. In ESPHome, this can be done by adding frequency: 15kHz
to the I2C configuration section.
💻 Code Examples
Below you can find code examples of AGS10 Sensor with ESP32 in several frameworks:
If you encounter issues while using the AGS10 Sensor, check the Common Issues Troubleshooting Guide.
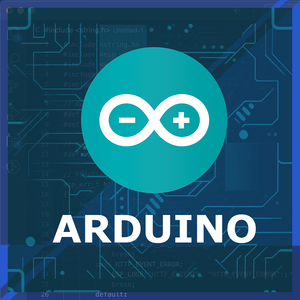
ESP32 AGS10 Arduino IDE Code Example
Fill in your main
Arduino IDE sketch file with the following code to use the AGS10 Sensor:
#include <Wire.h>
#define I2C_ADDRESS 0xXX // Replace with the AGS10 I2C address
#define SDA_PIN 21 // I2C SDA pin for ESP32
#define SCL_PIN 22 // I2C SCL pin for ESP32
void setup() {
Wire.begin(SDA_PIN, SCL_PIN);
Serial.begin(115200);
Serial.println("AGS10 I2C Test");
}
void loop() {
Wire.beginTransmission(I2C_ADDRESS);
Wire.write(0x00); // Example register or command
Wire.endTransmission();
Wire.requestFrom(I2C_ADDRESS, 2);
if (Wire.available() == 2) {
int highByte = Wire.read();
int lowByte = Wire.read();
int sensorValue = (highByte << 8) | lowByte;
Serial.print("Sensor Value: ");
Serial.println(sensorValue);
}
delay(1000);
}
This Arduino code demonstrates how to interact with the AGS10 sensor via I2C communication. It initializes the I2C interface using specified SDA and SCL pins, then communicates with the sensor by sending a command and requesting two bytes of data. The received high and low bytes are combined into a 16-bit integer to represent the sensor's value, which is printed to the Serial Monitor. The loop repeats every second, providing regular sensor updates.
Connect your ESP32 to your computer via a USB cable, Ensure the correct Board and Port are selected under Tools, Click the "Upload" button in the Arduino IDE to compile and upload the code to your ESP32.
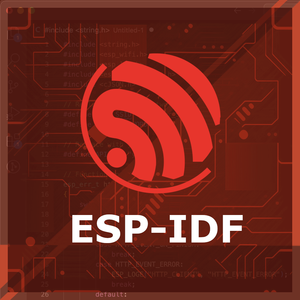
ESP32 AGS10 ESP-IDF Code ExampleExample in Espressif IoT Framework (ESP-IDF)
If you're using ESP-IDF to work with the AGS10 Sensor, here's how you can set it up and read data from the sensor. Fill in this code in the main
ESP-IDF file:
#include <stdio.h>
#include "driver/i2c.h"
#define I2C_MASTER_SCL_IO 22 // GPIO for SCL
#define I2C_MASTER_SDA_IO 21 // GPIO for SDA
#define I2C_MASTER_NUM I2C_NUM_1
#define I2C_MASTER_FREQ_HZ 100000
void app_main() {
i2c_config_t conf;
conf.mode = I2C_MODE_MASTER;
conf.sda_io_num = I2C_MASTER_SDA_IO;
conf.scl_io_num = I2C_MASTER_SCL_IO;
conf.sda_pullup_en = GPIO_PULLUP_ENABLE;
conf.scl_pullup_en = GPIO_PULLUP_ENABLE;
conf.master.clk_speed = I2C_MASTER_FREQ_HZ;
i2c_param_config(I2C_MASTER_NUM, &conf);
i2c_driver_install(I2C_MASTER_NUM, conf.mode, 0, 0, 0);
// Add code to read from AGS10 via I2C
}
This ESP-IDF code initializes the I2C master interface on an ESP32, configuring the SDA and SCL pins along with pull-up resistors and clock speed. The i2c_param_config()
and i2c_driver_install()
functions set up the I2C master for communication. You can add commands to communicate with the AGS10 sensor by sending and receiving I2C data after the initialization.
Update the I2C pins (I2C_MASTER_SDA_IO
and I2C_MASTER_SCL_IO
) to match your ESP32 hardware setup, Use idf.py build to compile the project, Use idf.py flash to upload the code to your ESP32.
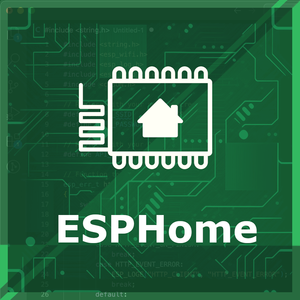
ESP32 AGS10 ESPHome Code Example
Fill in this configuration in your ESPHome YAML configuration file (example.yml
) to integrate the AGS10 Sensor
sensor:
- platform: ags10
tvoc:
name: TVOC
Upload this code to your ESP32 using the ESPHome dashboard or the esphome run
command.
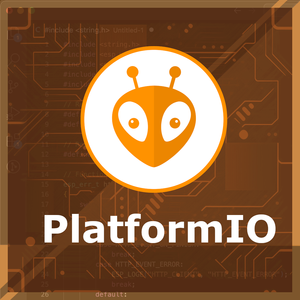
ESP32 AGS10 PlatformIO Code Example
For PlatformIO, make sure to configure the platformio.ini
file with the appropriate environment and libraries, and then proceed with the code.
Configure platformio.ini
First, your platformio.ini
should look like below. You might need to include some libraries as shown. Make sure to change the board to your ESP32:
[env:esp32]
platform = espressif32
board = esp32dev
framework = espidf
monitor_speed = 115200
ESP32 AGS10 PlatformIO Example Code
Write this code in your PlatformIO project under the src/main.cpp
file to use the AGS10 Sensor:
#include <Wire.h>
#define I2C_ADDRESS 0xXX // Replace with the AGS10 I2C address
#define SDA_PIN 21 // I2C SDA pin for ESP32
#define SCL_PIN 22 // I2C SCL pin for ESP32
void setup() {
Wire.begin(SDA_PIN, SCL_PIN);
Serial.begin(115200);
Serial.println("AGS10 I2C Test");
}
void loop() {
Wire.beginTransmission(I2C_ADDRESS);
Wire.write(0x00); // Example register or command
Wire.endTransmission();
Wire.requestFrom(I2C_ADDRESS, 2);
if (Wire.available() == 2) {
int highByte = Wire.read();
int lowByte = Wire.read();
int sensorValue = (highByte << 8) | lowByte;
Serial.print("Sensor Value: ");
Serial.println(sensorValue);
}
delay(1000);
}
This code is the same as the Arduino code but is intended for use with the PlatformIO environment. It initializes the I2C communication with the AGS10 sensor, reads data, and prints it to the Serial Monitor. Ensure the PlatformIO environment is correctly set up with the specified settings in the platformio.ini
file.
Upload the code to your ESP32 using the PlatformIO "Upload" button in your IDE or the pio run --target upload
command.
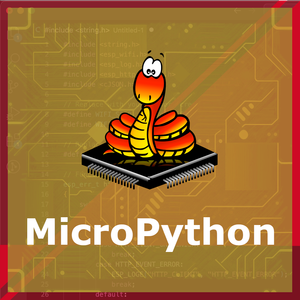
ESP32 AGS10 MicroPython Code Example
Fill in this script in your MicroPython main.py file (main.py
) to integrate the AGS10 Sensor with your ESP32.
from machine import I2C, Pin
from time import sleep
# Initialize I2C
i2c = I2C(0, scl=Pin(22), sda=Pin(21))
address = 0xXX # Replace with the AGS10 I2C address
while True:
# Write command and read data
i2c.writeto(address, b'\x00') # Example register or command
data = i2c.readfrom(address, 2)
# Combine high and low bytes into a 16-bit value
sensor_value = (data[0] << 8) | data[1]
print(f"Sensor Value: {sensor_value}")
sleep(1)
This MicroPython script uses the I2C protocol to communicate with the AGS10 sensor. It initializes the I2C instance with specified SDA and SCL pins, sends a command to the sensor, and reads two bytes of data. The received bytes are combined into a 16-bit integer to represent the sensor's value, which is printed to the console every second. Replace the placeholder I2C address with the correct address for your AGS10 sensor.
Upload this code to your ESP32 using a MicroPython-compatible IDE, such as Thonny, uPyCraft, or tools like ampy
.
Conclusion
We went through technical specifications of AGS10 Sensor, its pinout, connection with ESP32 and AGS10 Sensor code examples with Arduino IDE, ESP-IDF, ESPHome and PlatformIO.